Tip
An interactive online version of this notebook is available, which can be
accessed via
Alternatively, you may download this notebook and run it offline.
Attention
You are viewing this notebook on the latest version of the documentation, where these notebooks may not be compatible with the stable release of PyBaMM since they can contain features that are not yet released. We recommend viewing these notebooks from the stable version of the documentation. To install the latest version of PyBaMM that is compatible with the latest notebooks, build PyBaMM from source.
Simulating long experiments#
This notebook introduces functionality for simulating experiments over hundreds or even thousands of cycles.
[1]:
%pip install "pybamm[plot,cite]" -q # install PyBaMM if it is not installed
import pybamm
import matplotlib.pyplot as plt
Note: you may need to restart the kernel to use updated packages.
Simulating long experiments#
In the interest of simplicity and running time, we consider a SPM with SEI effects leading to linear degradation, with parameter values chosen so that the capacity fades by 20% in just a few cycles
[2]:
parameter_values = pybamm.ParameterValues("Mohtat2020")
parameter_values.update({"SEI kinetic rate constant [m.s-1]": 1e-14})
spm = pybamm.lithium_ion.SPM({"SEI": "ec reaction limited"})
We initialize the concentration in each electrode at 100% State of Charge
[3]:
# Calculate stoichiometries at 100% SOC
parameter_values.set_initial_stoichiometries(1);
We can now simulate a single CCCV cycle using the Experiment
class (see this notebook for more details)
[4]:
pybamm.set_logging_level("NOTICE")
experiment = pybamm.Experiment(
[
(
"Discharge at 1C until 3V",
"Rest for 1 hour",
"Charge at 1C until 4.2V",
"Hold at 4.2V until C/50",
)
]
)
sim = pybamm.Simulation(spm, experiment=experiment, parameter_values=parameter_values)
sol = sim.solve()
2022-12-04 21:24:29.130 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/1 (24.500 us elapsed) --------------------
2022-12-04 21:24:29.131 - [NOTICE] callbacks.on_step_start(182): Cycle 1/1, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:29.172 - [NOTICE] callbacks.on_step_start(182): Cycle 1/1, step 2/4: Rest for 1 hour
2022-12-04 21:24:29.199 - [NOTICE] callbacks.on_step_start(182): Cycle 1/1, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:29.237 - [NOTICE] callbacks.on_step_start(182): Cycle 1/1, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:29.372 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 241.861 ms
Alternatively, we can simulate many CCCV cycles. Here we simulate either 100 cycles or until the capacity is 80% of the initial capacity, whichever is first. The capacity is calculated by the eSOH model
[5]:
experiment = pybamm.Experiment(
[
(
"Discharge at 1C until 3V",
"Rest for 1 hour",
"Charge at 1C until 4.2V",
"Hold at 4.2V until C/50",
)
]
* 500,
termination="80% capacity",
)
sim = pybamm.Simulation(spm, experiment=experiment, parameter_values=parameter_values)
sol = sim.solve()
2022-12-04 21:24:30.209 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/500 (25.250 us elapsed) --------------------
2022-12-04 21:24:30.209 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:30.248 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:30.275 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:30.315 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:30.460 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.941 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:30.460 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/500 (251.525 ms elapsed) --------------------
2022-12-04 21:24:30.461 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:30.486 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:30.501 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:30.523 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:30.566 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.913 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:30.567 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/500 (357.953 ms elapsed) --------------------
2022-12-04 21:24:30.567 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:30.593 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:30.607 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:30.630 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:30.674 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.886 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:30.675 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/500 (465.886 ms elapsed) --------------------
2022-12-04 21:24:30.675 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:30.700 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:30.713 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:30.737 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:30.781 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.859 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:30.782 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/500 (573.195 ms elapsed) --------------------
2022-12-04 21:24:30.782 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:30.807 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:30.821 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:30.845 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:30.894 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.833 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:30.895 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/500 (686.094 ms elapsed) --------------------
2022-12-04 21:24:30.895 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:30.916 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:30.930 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:30.949 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:30.996 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.807 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:30.996 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/500 (787.595 ms elapsed) --------------------
2022-12-04 21:24:30.997 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.018 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.032 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.051 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.099 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.781 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.100 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/500 (891.219 ms elapsed) --------------------
2022-12-04 21:24:31.100 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.120 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.134 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.153 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.200 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.756 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.201 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/500 (992.032 ms elapsed) --------------------
2022-12-04 21:24:31.201 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.225 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.240 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.263 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.313 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.732 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.313 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/500 (1.104 s elapsed) --------------------
2022-12-04 21:24:31.313 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.337 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.351 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.372 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.420 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.708 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.421 - [NOTICE] callbacks.on_cycle_start(174): Cycle 11/500 (1.212 s elapsed) --------------------
2022-12-04 21:24:31.421 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.443 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.458 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.483 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.539 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.684 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.540 - [NOTICE] callbacks.on_cycle_start(174): Cycle 12/500 (1.331 s elapsed) --------------------
2022-12-04 21:24:31.542 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.565 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.579 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.597 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.644 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.660 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.645 - [NOTICE] callbacks.on_cycle_start(174): Cycle 13/500 (1.436 s elapsed) --------------------
2022-12-04 21:24:31.645 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.665 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.680 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.700 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.751 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.637 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.751 - [NOTICE] callbacks.on_cycle_start(174): Cycle 14/500 (1.543 s elapsed) --------------------
2022-12-04 21:24:31.752 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.774 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.788 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.807 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:31.938 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.614 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:31.938 - [NOTICE] callbacks.on_cycle_start(174): Cycle 15/500 (1.729 s elapsed) --------------------
2022-12-04 21:24:31.939 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:31.959 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:31.972 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:31.991 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.045 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.592 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.046 - [NOTICE] callbacks.on_cycle_start(174): Cycle 16/500 (1.837 s elapsed) --------------------
2022-12-04 21:24:32.046 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.068 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.082 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.103 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.153 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.569 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.154 - [NOTICE] callbacks.on_cycle_start(174): Cycle 17/500 (1.945 s elapsed) --------------------
2022-12-04 21:24:32.154 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.179 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.193 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.215 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.267 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.548 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.268 - [NOTICE] callbacks.on_cycle_start(174): Cycle 18/500 (2.059 s elapsed) --------------------
2022-12-04 21:24:32.268 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.290 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.304 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.326 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.378 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.526 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.379 - [NOTICE] callbacks.on_cycle_start(174): Cycle 19/500 (2.170 s elapsed) --------------------
2022-12-04 21:24:32.380 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.403 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.417 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.436 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.489 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.505 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.489 - [NOTICE] callbacks.on_cycle_start(174): Cycle 20/500 (2.281 s elapsed) --------------------
2022-12-04 21:24:32.490 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.508 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.522 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.540 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.594 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.484 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.595 - [NOTICE] callbacks.on_cycle_start(174): Cycle 21/500 (2.386 s elapsed) --------------------
2022-12-04 21:24:32.595 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.615 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.630 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.649 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.705 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.463 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.706 - [NOTICE] callbacks.on_cycle_start(174): Cycle 22/500 (2.497 s elapsed) --------------------
2022-12-04 21:24:32.707 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.728 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.742 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.762 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.816 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.442 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.817 - [NOTICE] callbacks.on_cycle_start(174): Cycle 23/500 (2.608 s elapsed) --------------------
2022-12-04 21:24:32.817 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.840 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.855 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.876 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:32.932 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.422 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:32.932 - [NOTICE] callbacks.on_cycle_start(174): Cycle 24/500 (2.724 s elapsed) --------------------
2022-12-04 21:24:32.933 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:32.954 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:32.968 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:32.998 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.056 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.402 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.056 - [NOTICE] callbacks.on_cycle_start(174): Cycle 25/500 (2.848 s elapsed) --------------------
2022-12-04 21:24:33.057 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.078 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.093 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.114 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.166 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.382 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.167 - [NOTICE] callbacks.on_cycle_start(174): Cycle 26/500 (2.958 s elapsed) --------------------
2022-12-04 21:24:33.167 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.188 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.203 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.224 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.279 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.362 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.280 - [NOTICE] callbacks.on_cycle_start(174): Cycle 27/500 (3.071 s elapsed) --------------------
2022-12-04 21:24:33.280 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.302 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.316 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.335 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.391 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.343 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.392 - [NOTICE] callbacks.on_cycle_start(174): Cycle 28/500 (3.183 s elapsed) --------------------
2022-12-04 21:24:33.392 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.415 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.429 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.448 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.503 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.324 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.504 - [NOTICE] callbacks.on_cycle_start(174): Cycle 29/500 (3.295 s elapsed) --------------------
2022-12-04 21:24:33.505 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.525 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.539 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.557 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.612 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.305 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.613 - [NOTICE] callbacks.on_cycle_start(174): Cycle 30/500 (3.404 s elapsed) --------------------
2022-12-04 21:24:33.613 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.631 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.645 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.665 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.724 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.286 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.724 - [NOTICE] callbacks.on_cycle_start(174): Cycle 31/500 (3.516 s elapsed) --------------------
2022-12-04 21:24:33.725 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.748 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.766 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.788 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.847 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.267 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.848 - [NOTICE] callbacks.on_cycle_start(174): Cycle 32/500 (3.639 s elapsed) --------------------
2022-12-04 21:24:33.848 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.871 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:33.894 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:33.914 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:33.972 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.249 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:33.972 - [NOTICE] callbacks.on_cycle_start(174): Cycle 33/500 (3.763 s elapsed) --------------------
2022-12-04 21:24:33.972 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:33.995 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.010 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.031 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.091 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.231 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.092 - [NOTICE] callbacks.on_cycle_start(174): Cycle 34/500 (3.883 s elapsed) --------------------
2022-12-04 21:24:34.092 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.114 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.128 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.149 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.208 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.213 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.209 - [NOTICE] callbacks.on_cycle_start(174): Cycle 35/500 (4.000 s elapsed) --------------------
2022-12-04 21:24:34.209 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.231 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.246 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.263 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.420 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.195 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.421 - [NOTICE] callbacks.on_cycle_start(174): Cycle 36/500 (4.212 s elapsed) --------------------
2022-12-04 21:24:34.421 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.444 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.458 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.475 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.535 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.177 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.536 - [NOTICE] callbacks.on_cycle_start(174): Cycle 37/500 (4.327 s elapsed) --------------------
2022-12-04 21:24:34.536 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.558 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.573 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.590 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.650 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.160 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.650 - [NOTICE] callbacks.on_cycle_start(174): Cycle 38/500 (4.442 s elapsed) --------------------
2022-12-04 21:24:34.651 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.668 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.682 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.700 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.763 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.143 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.763 - [NOTICE] callbacks.on_cycle_start(174): Cycle 39/500 (4.555 s elapsed) --------------------
2022-12-04 21:24:34.764 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.782 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.797 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.814 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.875 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.126 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.876 - [NOTICE] callbacks.on_cycle_start(174): Cycle 40/500 (4.667 s elapsed) --------------------
2022-12-04 21:24:34.876 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:34.895 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:34.910 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:34.931 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:34.990 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.109 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:34.991 - [NOTICE] callbacks.on_cycle_start(174): Cycle 41/500 (4.782 s elapsed) --------------------
2022-12-04 21:24:34.991 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.009 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.026 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.046 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.109 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.092 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.110 - [NOTICE] callbacks.on_cycle_start(174): Cycle 42/500 (4.901 s elapsed) --------------------
2022-12-04 21:24:35.110 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.130 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.144 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.163 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.225 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.075 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.225 - [NOTICE] callbacks.on_cycle_start(174): Cycle 43/500 (5.016 s elapsed) --------------------
2022-12-04 21:24:35.225 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.246 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.260 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.281 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.341 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.059 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.342 - [NOTICE] callbacks.on_cycle_start(174): Cycle 44/500 (5.133 s elapsed) --------------------
2022-12-04 21:24:35.342 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.364 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.383 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.403 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.462 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.042 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.463 - [NOTICE] callbacks.on_cycle_start(174): Cycle 45/500 (5.254 s elapsed) --------------------
2022-12-04 21:24:35.463 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.484 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.498 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.514 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.577 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.026 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.584 - [NOTICE] callbacks.on_cycle_start(174): Cycle 46/500 (5.376 s elapsed) --------------------
2022-12-04 21:24:35.593 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.633 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.659 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.675 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.740 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.010 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.741 - [NOTICE] callbacks.on_cycle_start(174): Cycle 47/500 (5.532 s elapsed) --------------------
2022-12-04 21:24:35.741 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.769 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.784 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.800 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.864 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.994 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.864 - [NOTICE] callbacks.on_cycle_start(174): Cycle 48/500 (5.655 s elapsed) --------------------
2022-12-04 21:24:35.864 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:35.881 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:35.896 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:35.914 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:35.983 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.978 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:35.983 - [NOTICE] callbacks.on_cycle_start(174): Cycle 49/500 (5.774 s elapsed) --------------------
2022-12-04 21:24:35.983 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:36.000 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:36.015 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:36.039 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:36.104 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.962 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:36.105 - [NOTICE] callbacks.on_cycle_start(174): Cycle 50/500 (5.896 s elapsed) --------------------
2022-12-04 21:24:36.105 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:36.123 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:36.140 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:36.160 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:36.227 - [NOTICE] callbacks.on_cycle_end(201): Stopping experiment since capacity (3.947 Ah) is below stopping capacity (3.952 Ah).
2022-12-04 21:24:36.229 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 6.019 s
Summary variables#
We can plot standard variables like the current and voltage, but it isn’t very instructive on these timescales
[6]:
sol.plot(["Current [A]", "Voltage [V]"])
[6]:
<pybamm.plotting.quick_plot.QuickPlot at 0x170561fd0>
Instead, we plot “summary variables”, which show how the battery degrades over time by various metrics. Some of the variables also have “Change in …”, which is how much that variable changes over each cycle. This can be achieved by using plot_summary_variables
method of pybamm, which can also be used to compare “summary variables” extracted from 2 or more solutions.
[7]:
sorted(sol.summary_variables.keys())
[7]:
['Capacity [A.h]',
'Change in local ECM resistance [Ohm]',
'Change in loss of active material in negative electrode [%]',
'Change in loss of active material in positive electrode [%]',
'Change in loss of capacity to SEI [A.h]',
'Change in loss of capacity to SEI on cracks [A.h]',
'Change in loss of capacity to lithium plating [A.h]',
'Change in loss of lithium inventory [%]',
'Change in loss of lithium inventory, including electrolyte [%]',
'Change in loss of lithium to SEI [mol]',
'Change in loss of lithium to SEI on cracks [mol]',
'Change in loss of lithium to lithium plating [mol]',
'Change in negative electrode capacity [A.h]',
'Change in positive electrode capacity [A.h]',
'Change in throughput capacity [A.h]',
'Change in throughput energy [W.h]',
'Change in time [h]',
'Change in time [s]',
'Change in total capacity lost to side reactions [A.h]',
'Change in total lithium [mol]',
'Change in total lithium in electrolyte [mol]',
'Change in total lithium in negative electrode [mol]',
'Change in total lithium in particles [mol]',
'Change in total lithium in positive electrode [mol]',
'Change in total lithium lost [mol]',
'Change in total lithium lost from electrolyte [mol]',
'Change in total lithium lost from particles [mol]',
'Change in total lithium lost to side reactions [mol]',
'Cycle number',
'Local ECM resistance [Ohm]',
'Loss of active material in negative electrode [%]',
'Loss of active material in positive electrode [%]',
'Loss of capacity to SEI [A.h]',
'Loss of capacity to SEI on cracks [A.h]',
'Loss of capacity to lithium plating [A.h]',
'Loss of lithium inventory [%]',
'Loss of lithium inventory, including electrolyte [%]',
'Loss of lithium to SEI [mol]',
'Loss of lithium to SEI on cracks [mol]',
'Loss of lithium to lithium plating [mol]',
'Maximum measured discharge capacity [A.h]',
'Maximum voltage [V]',
'Measured capacity [A.h]',
'Minimum measured discharge capacity [A.h]',
'Minimum voltage [V]',
'Negative electrode capacity [A.h]',
'Negative electrode excess capacity ratio',
'Positive electrode capacity [A.h]',
'Positive electrode excess capacity ratio',
'Q',
'Q_Li',
'Q_n',
'Q_n * (x_100 - x_0)',
'Q_p',
'Q_p * (y_0 - y_100)',
'Throughput capacity [A.h]',
'Throughput energy [W.h]',
'Time [h]',
'Time [s]',
'Total capacity lost to side reactions [A.h]',
'Total lithium [mol]',
'Total lithium in electrolyte [mol]',
'Total lithium in negative electrode [mol]',
'Total lithium in particles [mol]',
'Total lithium in positive electrode [mol]',
'Total lithium lost [mol]',
'Total lithium lost from electrolyte [mol]',
'Total lithium lost from particles [mol]',
'Total lithium lost to side reactions [mol]',
'Un(x_0)',
'Un(x_100)',
'Up(y_0)',
'Up(y_0) - Un(x_0)',
'Up(y_100)',
'Up(y_100) - Un(x_100)',
'n_Li',
'x_0',
'x_100',
'x_100 - x_0',
'y_0',
'y_0 - y_100',
'y_100']
The “summary variables” associated with a particular model can also be accessed as a list (which can then be edited) -
[8]:
spm.summary_variables
[8]:
['Time [s]',
'Time [h]',
'Throughput capacity [A.h]',
'Throughput energy [W.h]',
'Loss of lithium inventory [%]',
'Loss of lithium inventory, including electrolyte [%]',
'Total lithium [mol]',
'Total lithium in electrolyte [mol]',
'Total lithium in particles [mol]',
'Total lithium lost [mol]',
'Total lithium lost from particles [mol]',
'Total lithium lost from electrolyte [mol]',
'Loss of lithium to SEI [mol]',
'Loss of capacity to SEI [A.h]',
'Total lithium lost to side reactions [mol]',
'Total capacity lost to side reactions [A.h]',
'Local ECM resistance [Ohm]',
'Negative electrode capacity [A.h]',
'Loss of active material in negative electrode [%]',
'Total lithium in negative electrode [mol]',
'Loss of lithium to lithium plating [mol]',
'Loss of capacity to lithium plating [A.h]',
'Loss of lithium to SEI on cracks [mol]',
'Loss of capacity to SEI on cracks [A.h]',
'Positive electrode capacity [A.h]',
'Loss of active material in positive electrode [%]',
'Total lithium in positive electrode [mol]']
Here the only degradation mechanism is one that causes loss of lithium, so we don’t see loss of active material
[9]:
pybamm.plot_summary_variables(sol)
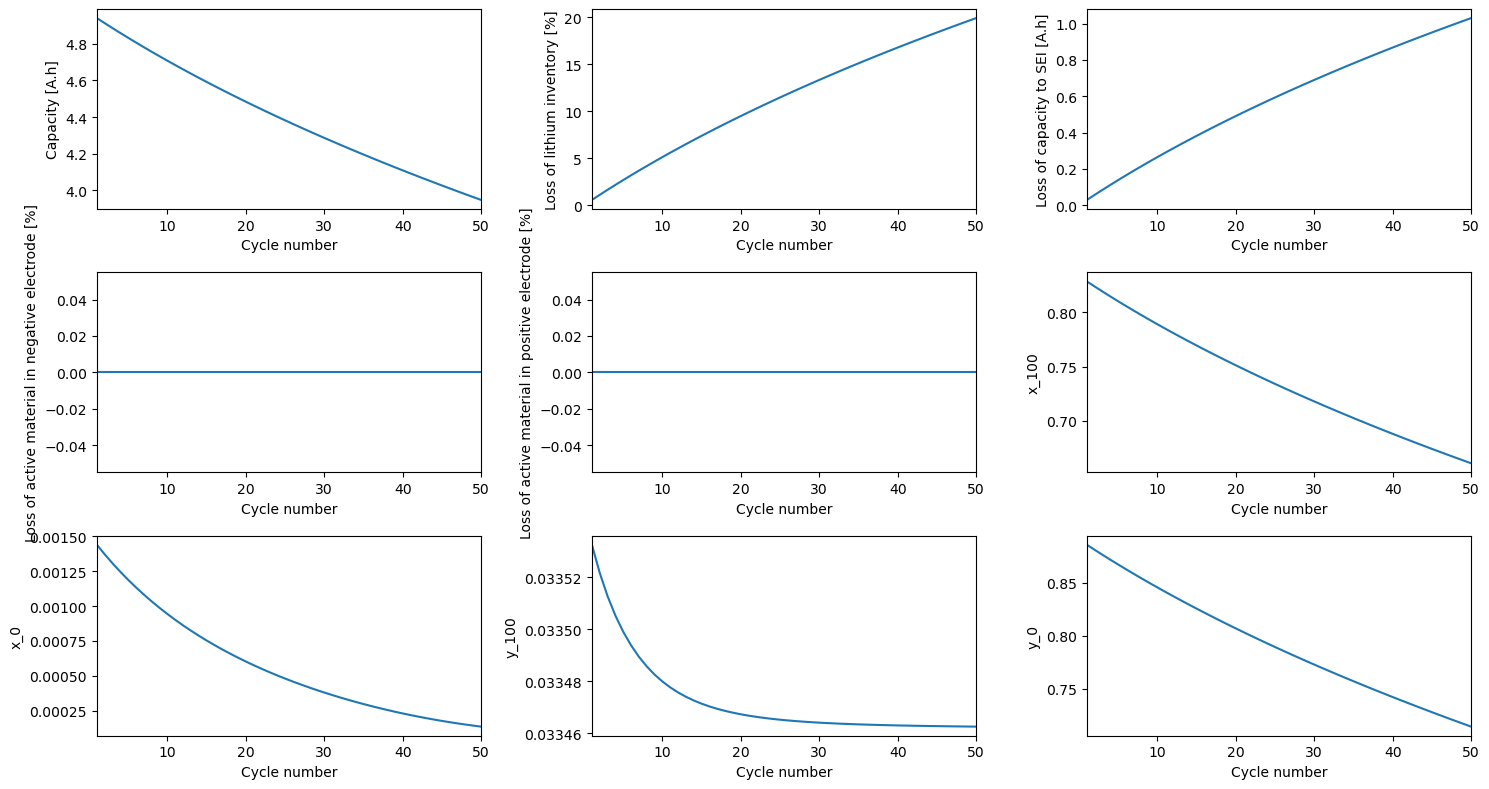
[9]:
array([[<AxesSubplot:xlabel='Cycle number', ylabel='Capacity [A.h]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of lithium inventory [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of capacity to SEI [A.h]'>],
[<AxesSubplot:xlabel='Cycle number', ylabel='Loss of active material in negative electrode [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of active material in positive electrode [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='x_100'>],
[<AxesSubplot:xlabel='Cycle number', ylabel='x_0'>,
<AxesSubplot:xlabel='Cycle number', ylabel='y_100'>,
<AxesSubplot:xlabel='Cycle number', ylabel='y_0'>]], dtype=object)
To suggest additional summary variables, open an issue!
Choosing which cycles to save#
If the simulation contains thousands of cycles, saving each cycle in RAM might not be possible. To get around this, we can use save_at_cycles
. If this is an integer n
, every nth cycle is saved. If this is a list, all the cycles in the list are saved. The first cycle is always saved.
[10]:
# With integer
sol_int = sim.solve(save_at_cycles=5)
# With list
sol_list = sim.solve(save_at_cycles=[30, 45, 55])
2022-12-04 21:24:37.738 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/500 (18.250 us elapsed) --------------------
2022-12-04 21:24:37.739 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:37.779 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:37.793 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:37.813 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:37.855 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.941 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:37.855 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/500 (117.477 ms elapsed) --------------------
2022-12-04 21:24:37.856 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:37.879 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:37.892 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:37.912 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:37.951 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.913 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:37.952 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/500 (213.869 ms elapsed) --------------------
2022-12-04 21:24:37.952 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:37.977 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:37.991 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.013 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.056 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.886 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.057 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/500 (319.000 ms elapsed) --------------------
2022-12-04 21:24:38.058 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.094 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.115 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.154 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.204 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.859 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.204 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/500 (466.169 ms elapsed) --------------------
2022-12-04 21:24:38.205 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.232 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.249 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.273 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.327 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.833 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.328 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/500 (590.261 ms elapsed) --------------------
2022-12-04 21:24:38.329 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.352 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.369 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.389 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.442 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.807 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.442 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/500 (704.200 ms elapsed) --------------------
2022-12-04 21:24:38.443 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.464 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.478 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.498 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.545 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.781 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.546 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/500 (807.534 ms elapsed) --------------------
2022-12-04 21:24:38.546 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.569 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.584 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.603 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.650 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.756 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.650 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/500 (912.304 ms elapsed) --------------------
2022-12-04 21:24:38.651 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.673 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.688 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.709 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.754 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.732 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.755 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/500 (1.017 s elapsed) --------------------
2022-12-04 21:24:38.756 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.780 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.795 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.817 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.866 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.708 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.867 - [NOTICE] callbacks.on_cycle_start(174): Cycle 11/500 (1.129 s elapsed) --------------------
2022-12-04 21:24:38.867 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.890 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:38.905 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:38.928 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:38.972 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.684 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:38.973 - [NOTICE] callbacks.on_cycle_start(174): Cycle 12/500 (1.235 s elapsed) --------------------
2022-12-04 21:24:38.973 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:38.997 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.010 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.029 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.074 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.660 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.075 - [NOTICE] callbacks.on_cycle_start(174): Cycle 13/500 (1.337 s elapsed) --------------------
2022-12-04 21:24:39.075 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.094 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.109 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.128 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.174 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.637 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.175 - [NOTICE] callbacks.on_cycle_start(174): Cycle 14/500 (1.437 s elapsed) --------------------
2022-12-04 21:24:39.176 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.198 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.211 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.230 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.274 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.614 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.274 - [NOTICE] callbacks.on_cycle_start(174): Cycle 15/500 (1.536 s elapsed) --------------------
2022-12-04 21:24:39.275 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.295 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.309 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.328 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.377 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.592 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.378 - [NOTICE] callbacks.on_cycle_start(174): Cycle 16/500 (1.640 s elapsed) --------------------
2022-12-04 21:24:39.378 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.400 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.414 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.437 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.482 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.569 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.482 - [NOTICE] callbacks.on_cycle_start(174): Cycle 17/500 (1.744 s elapsed) --------------------
2022-12-04 21:24:39.483 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.504 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.518 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.542 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.588 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.548 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.589 - [NOTICE] callbacks.on_cycle_start(174): Cycle 18/500 (1.851 s elapsed) --------------------
2022-12-04 21:24:39.589 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.613 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.627 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.654 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.700 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.526 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.700 - [NOTICE] callbacks.on_cycle_start(174): Cycle 19/500 (1.962 s elapsed) --------------------
2022-12-04 21:24:39.701 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.723 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.736 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.755 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.799 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.505 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.799 - [NOTICE] callbacks.on_cycle_start(174): Cycle 20/500 (2.061 s elapsed) --------------------
2022-12-04 21:24:39.800 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.820 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.833 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.852 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.899 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.484 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.899 - [NOTICE] callbacks.on_cycle_start(174): Cycle 21/500 (2.161 s elapsed) --------------------
2022-12-04 21:24:39.900 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:39.919 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:39.933 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:39.952 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:39.997 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.463 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:39.997 - [NOTICE] callbacks.on_cycle_start(174): Cycle 22/500 (2.259 s elapsed) --------------------
2022-12-04 21:24:39.998 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.017 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.031 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.050 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.092 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.442 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.093 - [NOTICE] callbacks.on_cycle_start(174): Cycle 23/500 (2.355 s elapsed) --------------------
2022-12-04 21:24:40.093 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.114 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.127 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.148 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.194 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.422 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.195 - [NOTICE] callbacks.on_cycle_start(174): Cycle 24/500 (2.457 s elapsed) --------------------
2022-12-04 21:24:40.195 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.217 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.231 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.253 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.299 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.402 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.299 - [NOTICE] callbacks.on_cycle_start(174): Cycle 25/500 (2.561 s elapsed) --------------------
2022-12-04 21:24:40.300 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.322 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.337 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.358 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.413 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.382 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.413 - [NOTICE] callbacks.on_cycle_start(174): Cycle 26/500 (2.675 s elapsed) --------------------
2022-12-04 21:24:40.414 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.439 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.456 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.480 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.526 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.362 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.527 - [NOTICE] callbacks.on_cycle_start(174): Cycle 27/500 (2.789 s elapsed) --------------------
2022-12-04 21:24:40.529 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.553 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.570 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.589 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.635 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.343 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.635 - [NOTICE] callbacks.on_cycle_start(174): Cycle 28/500 (2.897 s elapsed) --------------------
2022-12-04 21:24:40.635 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.658 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.673 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.693 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.737 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.324 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.738 - [NOTICE] callbacks.on_cycle_start(174): Cycle 29/500 (3.000 s elapsed) --------------------
2022-12-04 21:24:40.738 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.757 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.772 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.790 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.831 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.305 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.832 - [NOTICE] callbacks.on_cycle_start(174): Cycle 30/500 (3.094 s elapsed) --------------------
2022-12-04 21:24:40.833 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.851 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.864 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.882 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:40.926 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.286 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:40.927 - [NOTICE] callbacks.on_cycle_start(174): Cycle 31/500 (3.189 s elapsed) --------------------
2022-12-04 21:24:40.927 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:40.946 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:40.960 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:40.980 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.022 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.267 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.023 - [NOTICE] callbacks.on_cycle_start(174): Cycle 32/500 (3.285 s elapsed) --------------------
2022-12-04 21:24:41.023 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.042 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.056 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.078 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.122 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.249 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.123 - [NOTICE] callbacks.on_cycle_start(174): Cycle 33/500 (3.385 s elapsed) --------------------
2022-12-04 21:24:41.123 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.146 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.159 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.179 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.226 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.231 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.226 - [NOTICE] callbacks.on_cycle_start(174): Cycle 34/500 (3.488 s elapsed) --------------------
2022-12-04 21:24:41.227 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.252 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.267 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.288 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.333 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.213 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.334 - [NOTICE] callbacks.on_cycle_start(174): Cycle 35/500 (3.596 s elapsed) --------------------
2022-12-04 21:24:41.334 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.356 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.371 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.389 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.435 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.195 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.436 - [NOTICE] callbacks.on_cycle_start(174): Cycle 36/500 (3.698 s elapsed) --------------------
2022-12-04 21:24:41.436 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.461 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.474 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.492 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.539 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.177 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.540 - [NOTICE] callbacks.on_cycle_start(174): Cycle 37/500 (3.802 s elapsed) --------------------
2022-12-04 21:24:41.540 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.562 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.575 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.594 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.640 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.160 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.641 - [NOTICE] callbacks.on_cycle_start(174): Cycle 38/500 (3.903 s elapsed) --------------------
2022-12-04 21:24:41.641 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.659 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.673 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.692 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.738 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.143 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.738 - [NOTICE] callbacks.on_cycle_start(174): Cycle 39/500 (4.000 s elapsed) --------------------
2022-12-04 21:24:41.739 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.758 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.773 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.791 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.840 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.126 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.843 - [NOTICE] callbacks.on_cycle_start(174): Cycle 40/500 (4.105 s elapsed) --------------------
2022-12-04 21:24:41.848 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.869 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:41.888 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:41.916 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:41.973 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.109 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:41.974 - [NOTICE] callbacks.on_cycle_start(174): Cycle 41/500 (4.236 s elapsed) --------------------
2022-12-04 21:24:41.975 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:41.994 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.009 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.030 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.073 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.092 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.074 - [NOTICE] callbacks.on_cycle_start(174): Cycle 42/500 (4.336 s elapsed) --------------------
2022-12-04 21:24:42.074 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.092 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.108 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.129 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.174 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.075 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.174 - [NOTICE] callbacks.on_cycle_start(174): Cycle 43/500 (4.436 s elapsed) --------------------
2022-12-04 21:24:42.175 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.196 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.211 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.231 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.272 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.059 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.273 - [NOTICE] callbacks.on_cycle_start(174): Cycle 44/500 (4.535 s elapsed) --------------------
2022-12-04 21:24:42.273 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.295 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.309 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.330 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.372 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.042 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.373 - [NOTICE] callbacks.on_cycle_start(174): Cycle 45/500 (4.635 s elapsed) --------------------
2022-12-04 21:24:42.373 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.396 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.410 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.427 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.475 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.026 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.476 - [NOTICE] callbacks.on_cycle_start(174): Cycle 46/500 (4.738 s elapsed) --------------------
2022-12-04 21:24:42.476 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.498 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.519 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.536 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.582 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.010 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.582 - [NOTICE] callbacks.on_cycle_start(174): Cycle 47/500 (4.844 s elapsed) --------------------
2022-12-04 21:24:42.582 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.603 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.617 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.634 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.678 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.994 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.679 - [NOTICE] callbacks.on_cycle_start(174): Cycle 48/500 (4.941 s elapsed) --------------------
2022-12-04 21:24:42.680 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.698 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.711 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.727 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.772 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.978 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.772 - [NOTICE] callbacks.on_cycle_start(174): Cycle 49/500 (5.034 s elapsed) --------------------
2022-12-04 21:24:42.773 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.789 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.804 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.824 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.871 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.962 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:42.871 - [NOTICE] callbacks.on_cycle_start(174): Cycle 50/500 (5.133 s elapsed) --------------------
2022-12-04 21:24:42.871 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:42.890 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:42.904 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:42.924 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:42.977 - [NOTICE] callbacks.on_cycle_end(201): Stopping experiment since capacity (3.947 Ah) is below stopping capacity (3.952 Ah).
2022-12-04 21:24:42.979 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 5.239 s
2022-12-04 21:24:42.979 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/500 (15.583 us elapsed) --------------------
2022-12-04 21:24:42.980 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.020 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.036 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.061 - [NOTICE] callbacks.on_step_start(182): Cycle 1/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.105 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.941 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.106 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/500 (126.469 ms elapsed) --------------------
2022-12-04 21:24:43.107 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.131 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.145 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.166 - [NOTICE] callbacks.on_step_start(182): Cycle 2/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.209 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.913 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.209 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/500 (229.755 ms elapsed) --------------------
2022-12-04 21:24:43.210 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.232 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.246 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.270 - [NOTICE] callbacks.on_step_start(182): Cycle 3/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.315 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.886 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.316 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/500 (336.229 ms elapsed) --------------------
2022-12-04 21:24:43.316 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.341 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.357 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.382 - [NOTICE] callbacks.on_step_start(182): Cycle 4/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.424 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.859 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.425 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/500 (445.575 ms elapsed) --------------------
2022-12-04 21:24:43.426 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.450 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.463 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.485 - [NOTICE] callbacks.on_step_start(182): Cycle 5/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.531 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.833 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.532 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/500 (552.278 ms elapsed) --------------------
2022-12-04 21:24:43.532 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.552 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.572 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.592 - [NOTICE] callbacks.on_step_start(182): Cycle 6/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.640 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.807 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.641 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/500 (661.270 ms elapsed) --------------------
2022-12-04 21:24:43.642 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.666 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.682 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.704 - [NOTICE] callbacks.on_step_start(182): Cycle 7/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.762 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.781 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.763 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/500 (783.208 ms elapsed) --------------------
2022-12-04 21:24:43.763 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.788 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.805 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.832 - [NOTICE] callbacks.on_step_start(182): Cycle 8/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:43.883 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.756 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:43.889 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/500 (909.409 ms elapsed) --------------------
2022-12-04 21:24:43.902 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:43.939 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:43.955 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:43.977 - [NOTICE] callbacks.on_step_start(182): Cycle 9/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.022 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.732 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.023 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/500 (1.043 s elapsed) --------------------
2022-12-04 21:24:44.023 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.046 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.065 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.087 - [NOTICE] callbacks.on_step_start(182): Cycle 10/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.132 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.708 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.132 - [NOTICE] callbacks.on_cycle_start(174): Cycle 11/500 (1.153 s elapsed) --------------------
2022-12-04 21:24:44.132 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.157 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.172 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.194 - [NOTICE] callbacks.on_step_start(182): Cycle 11/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.238 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.684 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.238 - [NOTICE] callbacks.on_cycle_start(174): Cycle 12/500 (1.259 s elapsed) --------------------
2022-12-04 21:24:44.239 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.263 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.277 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.296 - [NOTICE] callbacks.on_step_start(182): Cycle 12/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.342 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.660 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.343 - [NOTICE] callbacks.on_cycle_start(174): Cycle 13/500 (1.363 s elapsed) --------------------
2022-12-04 21:24:44.343 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.363 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.377 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.399 - [NOTICE] callbacks.on_step_start(182): Cycle 13/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.447 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.637 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.448 - [NOTICE] callbacks.on_cycle_start(174): Cycle 14/500 (1.468 s elapsed) --------------------
2022-12-04 21:24:44.448 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.470 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.484 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.504 - [NOTICE] callbacks.on_step_start(182): Cycle 14/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.550 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.614 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.551 - [NOTICE] callbacks.on_cycle_start(174): Cycle 15/500 (1.572 s elapsed) --------------------
2022-12-04 21:24:44.551 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.572 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.586 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.604 - [NOTICE] callbacks.on_step_start(182): Cycle 15/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.651 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.592 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.651 - [NOTICE] callbacks.on_cycle_start(174): Cycle 16/500 (1.672 s elapsed) --------------------
2022-12-04 21:24:44.651 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.674 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.690 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.720 - [NOTICE] callbacks.on_step_start(182): Cycle 16/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.770 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.569 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.772 - [NOTICE] callbacks.on_cycle_start(174): Cycle 17/500 (1.792 s elapsed) --------------------
2022-12-04 21:24:44.774 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.802 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.828 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.851 - [NOTICE] callbacks.on_step_start(182): Cycle 17/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:44.897 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.548 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:44.898 - [NOTICE] callbacks.on_cycle_start(174): Cycle 18/500 (1.918 s elapsed) --------------------
2022-12-04 21:24:44.898 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:44.922 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:44.938 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:44.961 - [NOTICE] callbacks.on_step_start(182): Cycle 18/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.008 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.526 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.009 - [NOTICE] callbacks.on_cycle_start(174): Cycle 19/500 (2.030 s elapsed) --------------------
2022-12-04 21:24:45.010 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.033 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.046 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.065 - [NOTICE] callbacks.on_step_start(182): Cycle 19/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.111 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.505 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.111 - [NOTICE] callbacks.on_cycle_start(174): Cycle 20/500 (2.132 s elapsed) --------------------
2022-12-04 21:24:45.112 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.131 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.145 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.163 - [NOTICE] callbacks.on_step_start(182): Cycle 20/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.212 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.484 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.213 - [NOTICE] callbacks.on_cycle_start(174): Cycle 21/500 (2.233 s elapsed) --------------------
2022-12-04 21:24:45.213 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.235 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.249 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.268 - [NOTICE] callbacks.on_step_start(182): Cycle 21/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.314 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.463 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.315 - [NOTICE] callbacks.on_cycle_start(174): Cycle 22/500 (2.335 s elapsed) --------------------
2022-12-04 21:24:45.315 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.336 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.350 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.370 - [NOTICE] callbacks.on_step_start(182): Cycle 22/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.415 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.442 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.416 - [NOTICE] callbacks.on_cycle_start(174): Cycle 23/500 (2.436 s elapsed) --------------------
2022-12-04 21:24:45.416 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.437 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.450 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.471 - [NOTICE] callbacks.on_step_start(182): Cycle 23/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.690 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.422 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.690 - [NOTICE] callbacks.on_cycle_start(174): Cycle 24/500 (2.711 s elapsed) --------------------
2022-12-04 21:24:45.691 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.715 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.729 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.750 - [NOTICE] callbacks.on_step_start(182): Cycle 24/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.795 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.402 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.795 - [NOTICE] callbacks.on_cycle_start(174): Cycle 25/500 (2.816 s elapsed) --------------------
2022-12-04 21:24:45.796 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.819 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.833 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.855 - [NOTICE] callbacks.on_step_start(182): Cycle 25/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:45.899 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.382 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:45.900 - [NOTICE] callbacks.on_cycle_start(174): Cycle 26/500 (2.920 s elapsed) --------------------
2022-12-04 21:24:45.900 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:45.923 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:45.939 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:45.963 - [NOTICE] callbacks.on_step_start(182): Cycle 26/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.006 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.362 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.006 - [NOTICE] callbacks.on_cycle_start(174): Cycle 27/500 (3.027 s elapsed) --------------------
2022-12-04 21:24:46.007 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.028 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.043 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.061 - [NOTICE] callbacks.on_step_start(182): Cycle 27/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.109 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.343 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.109 - [NOTICE] callbacks.on_cycle_start(174): Cycle 28/500 (3.130 s elapsed) --------------------
2022-12-04 21:24:46.110 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.134 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.147 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.165 - [NOTICE] callbacks.on_step_start(182): Cycle 28/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.209 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.324 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.210 - [NOTICE] callbacks.on_cycle_start(174): Cycle 29/500 (3.230 s elapsed) --------------------
2022-12-04 21:24:46.210 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.233 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.247 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.265 - [NOTICE] callbacks.on_step_start(182): Cycle 29/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.310 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.305 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.311 - [NOTICE] callbacks.on_cycle_start(174): Cycle 30/500 (3.332 s elapsed) --------------------
2022-12-04 21:24:46.312 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.332 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.346 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.364 - [NOTICE] callbacks.on_step_start(182): Cycle 30/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.411 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.286 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.411 - [NOTICE] callbacks.on_cycle_start(174): Cycle 31/500 (3.432 s elapsed) --------------------
2022-12-04 21:24:46.412 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.432 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.445 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.465 - [NOTICE] callbacks.on_step_start(182): Cycle 31/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.508 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.267 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.509 - [NOTICE] callbacks.on_cycle_start(174): Cycle 32/500 (3.529 s elapsed) --------------------
2022-12-04 21:24:46.509 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.528 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.546 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.566 - [NOTICE] callbacks.on_step_start(182): Cycle 32/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.612 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.249 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.613 - [NOTICE] callbacks.on_cycle_start(174): Cycle 33/500 (3.634 s elapsed) --------------------
2022-12-04 21:24:46.613 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.635 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.650 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.670 - [NOTICE] callbacks.on_step_start(182): Cycle 33/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.714 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.231 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.714 - [NOTICE] callbacks.on_cycle_start(174): Cycle 34/500 (3.735 s elapsed) --------------------
2022-12-04 21:24:46.715 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.736 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.749 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.769 - [NOTICE] callbacks.on_step_start(182): Cycle 34/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.814 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.213 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.814 - [NOTICE] callbacks.on_cycle_start(174): Cycle 35/500 (3.835 s elapsed) --------------------
2022-12-04 21:24:46.815 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.837 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.857 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:46.877 - [NOTICE] callbacks.on_step_start(182): Cycle 35/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:46.922 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.195 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:46.926 - [NOTICE] callbacks.on_cycle_start(174): Cycle 36/500 (3.947 s elapsed) --------------------
2022-12-04 21:24:46.928 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:46.952 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:46.986 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.020 - [NOTICE] callbacks.on_step_start(182): Cycle 36/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.094 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.177 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.095 - [NOTICE] callbacks.on_cycle_start(174): Cycle 37/500 (4.115 s elapsed) --------------------
2022-12-04 21:24:47.095 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.120 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.134 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.151 - [NOTICE] callbacks.on_step_start(182): Cycle 37/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.195 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.160 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.196 - [NOTICE] callbacks.on_cycle_start(174): Cycle 38/500 (4.216 s elapsed) --------------------
2022-12-04 21:24:47.196 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.216 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.233 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.252 - [NOTICE] callbacks.on_step_start(182): Cycle 38/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.296 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.143 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.297 - [NOTICE] callbacks.on_cycle_start(174): Cycle 39/500 (4.317 s elapsed) --------------------
2022-12-04 21:24:47.297 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.318 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.333 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.352 - [NOTICE] callbacks.on_step_start(182): Cycle 39/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.396 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.126 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.397 - [NOTICE] callbacks.on_cycle_start(174): Cycle 40/500 (4.417 s elapsed) --------------------
2022-12-04 21:24:47.397 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.418 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.432 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.450 - [NOTICE] callbacks.on_step_start(182): Cycle 40/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.493 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.109 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.494 - [NOTICE] callbacks.on_cycle_start(174): Cycle 41/500 (4.514 s elapsed) --------------------
2022-12-04 21:24:47.494 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.512 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.528 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.551 - [NOTICE] callbacks.on_step_start(182): Cycle 41/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.594 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.092 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.594 - [NOTICE] callbacks.on_cycle_start(174): Cycle 42/500 (4.615 s elapsed) --------------------
2022-12-04 21:24:47.594 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.613 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.627 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.649 - [NOTICE] callbacks.on_step_start(182): Cycle 42/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.693 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.075 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.693 - [NOTICE] callbacks.on_cycle_start(174): Cycle 43/500 (4.714 s elapsed) --------------------
2022-12-04 21:24:47.694 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.716 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.729 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.748 - [NOTICE] callbacks.on_step_start(182): Cycle 43/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.791 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.059 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.792 - [NOTICE] callbacks.on_cycle_start(174): Cycle 44/500 (4.813 s elapsed) --------------------
2022-12-04 21:24:47.793 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.817 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.835 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.854 - [NOTICE] callbacks.on_step_start(182): Cycle 44/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:47.899 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.042 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:47.900 - [NOTICE] callbacks.on_cycle_start(174): Cycle 45/500 (4.920 s elapsed) --------------------
2022-12-04 21:24:47.900 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:47.922 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:47.938 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:47.958 - [NOTICE] callbacks.on_step_start(182): Cycle 45/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:48.003 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.026 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:48.003 - [NOTICE] callbacks.on_cycle_start(174): Cycle 46/500 (5.024 s elapsed) --------------------
2022-12-04 21:24:48.004 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:48.027 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:48.041 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:48.060 - [NOTICE] callbacks.on_step_start(182): Cycle 46/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:48.117 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.010 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:48.122 - [NOTICE] callbacks.on_cycle_start(174): Cycle 47/500 (5.142 s elapsed) --------------------
2022-12-04 21:24:48.127 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:48.155 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:48.193 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:48.208 - [NOTICE] callbacks.on_step_start(182): Cycle 47/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:48.256 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.994 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:48.256 - [NOTICE] callbacks.on_cycle_start(174): Cycle 48/500 (5.277 s elapsed) --------------------
2022-12-04 21:24:48.257 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:48.274 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:48.290 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:48.309 - [NOTICE] callbacks.on_step_start(182): Cycle 48/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:48.356 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.978 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:48.357 - [NOTICE] callbacks.on_cycle_start(174): Cycle 49/500 (5.378 s elapsed) --------------------
2022-12-04 21:24:48.358 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:48.375 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:48.389 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:48.410 - [NOTICE] callbacks.on_step_start(182): Cycle 49/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:48.457 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 3.962 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:48.458 - [NOTICE] callbacks.on_cycle_start(174): Cycle 50/500 (5.478 s elapsed) --------------------
2022-12-04 21:24:48.458 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:48.478 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 2/4: Rest for 1 hour
2022-12-04 21:24:48.494 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:48.513 - [NOTICE] callbacks.on_step_start(182): Cycle 50/500, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:48.560 - [NOTICE] callbacks.on_cycle_end(201): Stopping experiment since capacity (3.947 Ah) is below stopping capacity (3.952 Ah).
2022-12-04 21:24:48.561 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 5.580 s
[11]:
sol_int.cycles
[11]:
[<pybamm.solvers.solution.Solution at 0x1729c8130>,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x17299b760>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172a40220>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172b05190>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172c5f2e0>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172d43730>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172bc1ac0>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172f10940>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x172ec28e0>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x1731078b0>,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x1731a2520>]
[12]:
sol_list.cycles
[12]:
[<pybamm.solvers.solution.Solution at 0x17331d670>,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x17330ef10>,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
None,
<pybamm.solvers.solution.Solution at 0x173482220>,
None,
None,
None,
None,
None]
For the cycles that are saved, you can plot as usual (note off-by-1 indexing)
[13]:
sol_list.cycles[44].plot(["Current [A]", "Voltage [V]"])
[13]:
<pybamm.plotting.quick_plot.QuickPlot at 0x172afde20>
[14]:
fig, ax = plt.subplots(1, 2, figsize=(10, 5))
for cycle in sol_int.cycles:
if cycle is not None:
t = cycle["Time [h]"].data - cycle["Time [h]"].data[0]
ax[0].plot(t, cycle["Current [A]"].data)
ax[0].set_xlabel("Time [h]")
ax[0].set_title("Current [A]")
ax[1].plot(t, cycle["Voltage [V]"].data)
ax[1].set_xlabel("Time [h]")
ax[1].set_title("Voltage [V]")
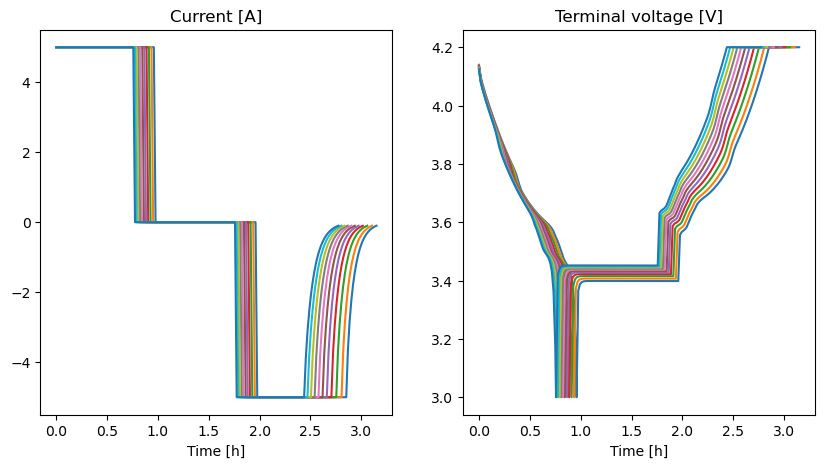
All summary variables are always available for every cycle, since these are much less memory-intensive
[15]:
pybamm.plot_summary_variables(sol_list)
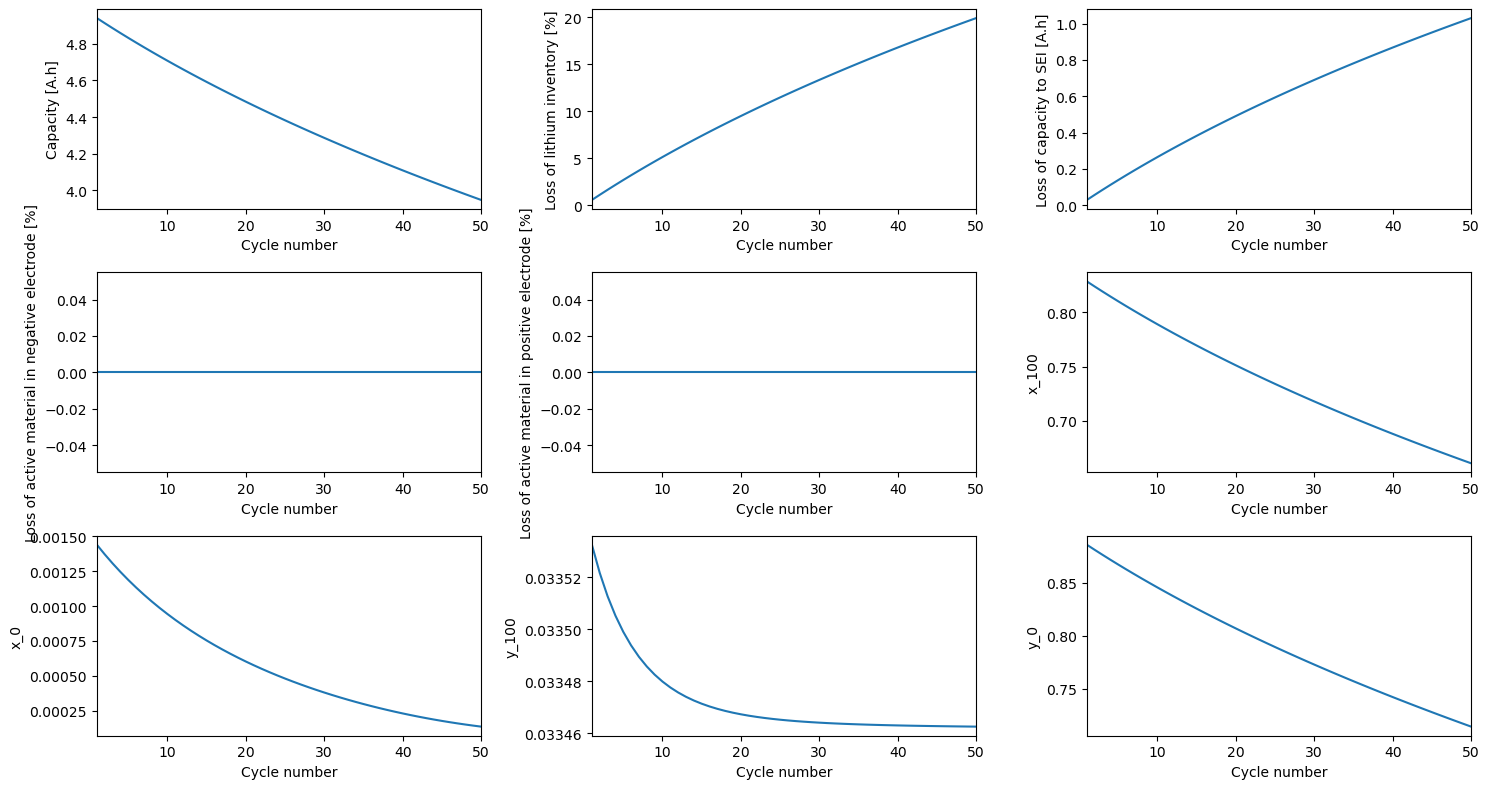
[15]:
array([[<AxesSubplot:xlabel='Cycle number', ylabel='Capacity [A.h]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of lithium inventory [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of capacity to SEI [A.h]'>],
[<AxesSubplot:xlabel='Cycle number', ylabel='Loss of active material in negative electrode [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of active material in positive electrode [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='x_100'>],
[<AxesSubplot:xlabel='Cycle number', ylabel='x_0'>,
<AxesSubplot:xlabel='Cycle number', ylabel='y_100'>,
<AxesSubplot:xlabel='Cycle number', ylabel='y_0'>]], dtype=object)
Starting solution#
A simulation can be performed iteratively by using the starting_solution
feature. For example, we first solve for 10 cycles
[16]:
experiment = pybamm.Experiment(
[
(
"Discharge at 1C until 3V",
"Rest for 1 hour",
"Charge at 1C until 4.2V",
"Hold at 4.2V until C/50",
)
]
* 10,
termination="80% capacity",
)
sim = pybamm.Simulation(spm, experiment=experiment, parameter_values=parameter_values)
sol = sim.solve()
2022-12-04 21:24:50.503 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/10 (22.792 us elapsed) --------------------
2022-12-04 21:24:50.503 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:50.544 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:50.572 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:50.613 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:50.754 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.941 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:50.754 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/10 (251.450 ms elapsed) --------------------
2022-12-04 21:24:50.755 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:50.779 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:50.800 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:50.825 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:50.868 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.913 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:50.869 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/10 (366.027 ms elapsed) --------------------
2022-12-04 21:24:50.869 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:50.893 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:50.909 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:50.934 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:50.988 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.886 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:50.989 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/10 (486.547 ms elapsed) --------------------
2022-12-04 21:24:50.990 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.016 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.031 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.055 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.103 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.859 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.104 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/10 (600.658 ms elapsed) --------------------
2022-12-04 21:24:51.104 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.128 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.143 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.171 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.230 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.833 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.230 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/10 (727.148 ms elapsed) --------------------
2022-12-04 21:24:51.230 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.253 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.267 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.288 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.337 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.807 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.338 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/10 (834.650 ms elapsed) --------------------
2022-12-04 21:24:51.338 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.361 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.381 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.401 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.449 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.781 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.450 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/10 (946.869 ms elapsed) --------------------
2022-12-04 21:24:51.450 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.470 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.484 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.503 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.551 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.756 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.552 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/10 (1.049 s elapsed) --------------------
2022-12-04 21:24:51.552 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.575 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.589 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.611 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.659 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.732 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.660 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/10 (1.157 s elapsed) --------------------
2022-12-04 21:24:51.660 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.682 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.695 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.717 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.766 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.708 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.767 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 1.263 s
If we give sol
as the starting solution this will then solve for the next 10 cycles
[17]:
sol2 = sim.solve(starting_solution=sol)
2022-12-04 21:24:51.827 - [NOTICE] callbacks.on_cycle_start(174): Cycle 11/20 (30.792 us elapsed) --------------------
2022-12-04 21:24:51.828 - [NOTICE] callbacks.on_step_start(182): Cycle 11/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.859 - [NOTICE] callbacks.on_step_start(182): Cycle 11/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:51.876 - [NOTICE] callbacks.on_step_start(182): Cycle 11/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:51.900 - [NOTICE] callbacks.on_step_start(182): Cycle 11/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:51.974 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.684 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:51.974 - [NOTICE] callbacks.on_cycle_start(174): Cycle 12/20 (147.399 ms elapsed) --------------------
2022-12-04 21:24:51.975 - [NOTICE] callbacks.on_step_start(182): Cycle 12/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:51.997 - [NOTICE] callbacks.on_step_start(182): Cycle 12/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.010 - [NOTICE] callbacks.on_step_start(182): Cycle 12/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.028 - [NOTICE] callbacks.on_step_start(182): Cycle 12/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.227 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.660 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.227 - [NOTICE] callbacks.on_cycle_start(174): Cycle 13/20 (400.113 ms elapsed) --------------------
2022-12-04 21:24:52.227 - [NOTICE] callbacks.on_step_start(182): Cycle 13/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.245 - [NOTICE] callbacks.on_step_start(182): Cycle 13/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.258 - [NOTICE] callbacks.on_step_start(182): Cycle 13/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.276 - [NOTICE] callbacks.on_step_start(182): Cycle 13/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.326 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.637 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.326 - [NOTICE] callbacks.on_cycle_start(174): Cycle 14/20 (498.938 ms elapsed) --------------------
2022-12-04 21:24:52.326 - [NOTICE] callbacks.on_step_start(182): Cycle 14/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.345 - [NOTICE] callbacks.on_step_start(182): Cycle 14/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.360 - [NOTICE] callbacks.on_step_start(182): Cycle 14/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.378 - [NOTICE] callbacks.on_step_start(182): Cycle 14/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.428 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.614 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.428 - [NOTICE] callbacks.on_cycle_start(174): Cycle 15/20 (601.364 ms elapsed) --------------------
2022-12-04 21:24:52.429 - [NOTICE] callbacks.on_step_start(182): Cycle 15/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.450 - [NOTICE] callbacks.on_step_start(182): Cycle 15/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.463 - [NOTICE] callbacks.on_step_start(182): Cycle 15/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.481 - [NOTICE] callbacks.on_step_start(182): Cycle 15/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.534 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.592 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.534 - [NOTICE] callbacks.on_cycle_start(174): Cycle 16/20 (707.075 ms elapsed) --------------------
2022-12-04 21:24:52.535 - [NOTICE] callbacks.on_step_start(182): Cycle 16/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.558 - [NOTICE] callbacks.on_step_start(182): Cycle 16/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.571 - [NOTICE] callbacks.on_step_start(182): Cycle 16/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.593 - [NOTICE] callbacks.on_step_start(182): Cycle 16/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.645 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.569 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.645 - [NOTICE] callbacks.on_cycle_start(174): Cycle 17/20 (818.355 ms elapsed) --------------------
2022-12-04 21:24:52.646 - [NOTICE] callbacks.on_step_start(182): Cycle 17/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.669 - [NOTICE] callbacks.on_step_start(182): Cycle 17/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.683 - [NOTICE] callbacks.on_step_start(182): Cycle 17/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.709 - [NOTICE] callbacks.on_step_start(182): Cycle 17/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.762 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.548 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.763 - [NOTICE] callbacks.on_cycle_start(174): Cycle 18/20 (935.547 ms elapsed) --------------------
2022-12-04 21:24:52.763 - [NOTICE] callbacks.on_step_start(182): Cycle 18/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.786 - [NOTICE] callbacks.on_step_start(182): Cycle 18/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.799 - [NOTICE] callbacks.on_step_start(182): Cycle 18/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.821 - [NOTICE] callbacks.on_step_start(182): Cycle 18/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.877 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.526 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.878 - [NOTICE] callbacks.on_cycle_start(174): Cycle 19/20 (1.051 s elapsed) --------------------
2022-12-04 21:24:52.878 - [NOTICE] callbacks.on_step_start(182): Cycle 19/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:52.900 - [NOTICE] callbacks.on_step_start(182): Cycle 19/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:52.914 - [NOTICE] callbacks.on_step_start(182): Cycle 19/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:52.933 - [NOTICE] callbacks.on_step_start(182): Cycle 19/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:52.988 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.505 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:52.988 - [NOTICE] callbacks.on_cycle_start(174): Cycle 20/20 (1.161 s elapsed) --------------------
2022-12-04 21:24:52.989 - [NOTICE] callbacks.on_step_start(182): Cycle 20/20, step 1/4: Discharge at 1C until 3V
2022-12-04 21:24:53.007 - [NOTICE] callbacks.on_step_start(182): Cycle 20/20, step 2/4: Rest for 1 hour
2022-12-04 21:24:53.020 - [NOTICE] callbacks.on_step_start(182): Cycle 20/20, step 3/4: Charge at 1C until 4.2V
2022-12-04 21:24:53.039 - [NOTICE] callbacks.on_step_start(182): Cycle 20/20, step 4/4: Hold at 4.2V until C/50
2022-12-04 21:24:53.094 - [NOTICE] callbacks.on_cycle_end(196): Capacity is now 4.484 Ah (originally 4.941 Ah, will stop at 3.952 Ah)
2022-12-04 21:24:53.095 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 1.267 s
We have now simulated 20 cycles
[18]:
len(sol2.cycles)
[18]:
20
References#
The relevant papers for this notebook are:
[19]:
pybamm.print_citations()
[1] Weilong Ai, Ludwig Kraft, Johannes Sturm, Andreas Jossen, and Billy Wu. Electrochemical thermal-mechanical modelling of stress inhomogeneity in lithium-ion pouch cells. Journal of The Electrochemical Society, 167(1):013512, 2019. doi:10.1149/2.0122001JES.
[2] Joel A. E. Andersson, Joris Gillis, Greg Horn, James B. Rawlings, and Moritz Diehl. CasADi – A software framework for nonlinear optimization and optimal control. Mathematical Programming Computation, 11(1):1–36, 2019. doi:10.1007/s12532-018-0139-4.
[3] Ferran Brosa Planella and W. Dhammika Widanage. Systematic derivation of a Single Particle Model with Electrolyte and Side Reactions (SPMe+SR) for degradation of lithium-ion batteries. Submitted for publication, ():, 2022. doi:.
[4] Rutooj Deshpande, Mark Verbrugge, Yang-Tse Cheng, John Wang, and Ping Liu. Battery cycle life prediction with coupled chemical degradation and fatigue mechanics. Journal of the Electrochemical Society, 159(10):A1730, 2012. doi:10.1149/2.049210jes.
[5] Charles R. Harris, K. Jarrod Millman, Stéfan J. van der Walt, Ralf Gommers, Pauli Virtanen, David Cournapeau, Eric Wieser, Julian Taylor, Sebastian Berg, Nathaniel J. Smith, and others. Array programming with NumPy. Nature, 585(7825):357–362, 2020. doi:10.1038/s41586-020-2649-2.
[6] Scott G. Marquis, Valentin Sulzer, Robert Timms, Colin P. Please, and S. Jon Chapman. An asymptotic derivation of a single particle model with electrolyte. Journal of The Electrochemical Society, 166(15):A3693–A3706, 2019. doi:10.1149/2.0341915jes.
[7] Peyman Mohtat, Suhak Lee, Jason B Siegel, and Anna G Stefanopoulou. Towards better estimability of electrode-specific state of health: decoding the cell expansion. Journal of Power Sources, 427:101–111, 2019.
[8] Peyman Mohtat, Suhak Lee, Valentin Sulzer, Jason B. Siegel, and Anna G. Stefanopoulou. Differential Expansion and Voltage Model for Li-ion Batteries at Practical Charging Rates. Journal of The Electrochemical Society, 167(11):110561, 2020. doi:10.1149/1945-7111/aba5d1.
[9] Valentin Sulzer, Scott G. Marquis, Robert Timms, Martin Robinson, and S. Jon Chapman. Python Battery Mathematical Modelling (PyBaMM). Journal of Open Research Software, 9(1):14, 2021. doi:10.5334/jors.309.
[10] Pauli Virtanen, Ralf Gommers, Travis E. Oliphant, Matt Haberland, Tyler Reddy, David Cournapeau, Evgeni Burovski, Pearu Peterson, Warren Weckesser, Jonathan Bright, and others. SciPy 1.0: fundamental algorithms for scientific computing in Python. Nature Methods, 17(3):261–272, 2020. doi:10.1038/s41592-019-0686-2.