Tip
An interactive online version of this notebook is available, which can be
accessed via
Alternatively, you may download this notebook and run it offline.
Attention
You are viewing this notebook on the latest version of the documentation, where these notebooks may not be compatible with the stable release of PyBaMM since they can contain features that are not yet released. We recommend viewing these notebooks from the stable version of the documentation. To install the latest version of PyBaMM that is compatible with the latest notebooks, build PyBaMM from source.
Comparisons using the BatchStudy class#
In this notebook, we will be going through the BatchStudy
class and will be discussing how different models, experiments, chemistries, etc. can be compared with each other using the same.
Comparing models#
We start by creating a simple script to compare SPM
, SPMe
and DFN
model with the default parameters.
[1]:
%pip install "pybamm[plot,cite]" -q # install PyBaMM if it is not installed
import pybamm
# loading up 3 models to compare
dfn = pybamm.lithium_ion.DFN()
spm = pybamm.lithium_ion.SPM()
spme = pybamm.lithium_ion.SPMe()
Note: you may need to restart the kernel to use updated packages.
The BatchStudy
class requires a dictionary of models, and all the default values for a given model are used if no additional parameter is passed in.
[2]:
models = {
"dfn": dfn,
"spm": spm,
"spme": spme,
}
# creating a BatchStudy object
batch_study = pybamm.BatchStudy(models=models)
# solving and plotting the comparison
batch_study.solve(t_eval=[0, 3600])
batch_study.plot()
[2]:
<pybamm.plotting.quick_plot.QuickPlot at 0x7ffdb8988b80>
BatchStudy
by default requires equal number of items in all the dictionaries passed, which can be changed by setting the value of permutations
to True
. When set True
, a cartesian product of all the available items is taken.
For example, here we pass 3 models but only 1 parameter value, hence it is necessary to set permutations
to True
. Here, the given parameter value is used for all the provided models.
[3]:
# passing parameter_values as a dictionary
parameter_values = {"Chen2020": pybamm.ParameterValues("Chen2020")}
# creating a BatchStudy object and solving the simulation
batch_study = pybamm.BatchStudy(
models=models, parameter_values=parameter_values, permutations=True
)
batch_study.solve(t_eval=[0, 3600])
batch_study.plot()
[3]:
<pybamm.plotting.quick_plot.QuickPlot at 0x7ffdcba8cfa0>
Comparing parameters#
BatchStudy
can also be used to compare different things (like affect of changing a parameter’s value) on a single model.
In the following cell, we compare different values of "Curent function [A]"
using the Single Paritcle Model with electrolyte
.
[4]:
model = {"spme": spme}
# populating a dictionary with 3 same parameter values
parameter_values = {
"Chen2020_1": pybamm.ParameterValues("Chen2020"),
"Chen2020_2": pybamm.ParameterValues("Chen2020"),
"Chen2020_3": pybamm.ParameterValues("Chen2020"),
}
# different values for "Current function [A]"
current_values = [4.5, 4.75, 5]
# changing the value of "Current function [A]" in all the parameter values present in the
# parameter_values dictionary
for _, v, current_value in zip(
parameter_values.keys(), parameter_values.values(), current_values
):
v["Current function [A]"] = current_value
# creating a BatchStudy object with permutations set to True to create a cartesian product
batch_study = pybamm.BatchStudy(
models=model, parameter_values=parameter_values, permutations=True
)
batch_study.solve(t_eval=[0, 3600])
# generating the required labels and plotting
labels = [f"Current function [A]: {current}" for current in current_values]
batch_study.plot(labels=labels)
[4]:
<pybamm.plotting.quick_plot.QuickPlot at 0x7ffdccc5bd30>
BatchStudy
also includes a create_gif
method which can be used to create a GIF of the simulation.
[5]:
# using less number of images in the example
# for a smoother GIF use more images
batch_study.create_gif(number_of_images=5, duration=0.2, output_filename="batch.gif")
Displaying the GIF using markdown-
Using experiments#
Experiments can also be specified for comparisons, and they are also passed as a dictionary (a dictionary of pybamm.Experiment
) in the BatchStudy
class.
In the next cell, we compare a single experiment, with a single model, but with a varied parameter value.
[6]:
pybamm.set_logging_level("NOTICE")
# using the cccv experiment with 10 cycles
cccv = pybamm.Experiment(
[
(
"Discharge at C/10 for 10 hours or until 3.3 V",
"Rest for 1 hour",
"Charge at 1 A until 4.1 V",
"Hold at 4.1 V until 50 mA",
"Rest for 1 hour",
)
]
* 10,
)
# creating the experiment dict
experiment = {"cccv": cccv}
# populating a dictionary with 3 same parameter values (Mohtat2020 chemistry)
parameter_values = {
"Mohtat2020_1": pybamm.ParameterValues("Mohtat2020"),
"Mohtat2020_2": pybamm.ParameterValues("Mohtat2020"),
"Mohtat2020_3": pybamm.ParameterValues("Mohtat2020"),
}
# different values for the parameter "Inner SEI open-circuit potential [V]"
inner_sei_oc_v_values = [2.0e-4, 2.7e-4, 3.4e-4]
# updating the value of "Inner SEI open-circuit potential [V]" in all the dictionary items
for _, v, inner_sei_oc_v in zip(
parameter_values.keys(), parameter_values.values(), inner_sei_oc_v_values
):
v.update(
{"Inner SEI open-circuit potential [V]": inner_sei_oc_v},
)
# creating a Single Particle Model with "electron-mitigation limited" SEI
model = {"spm": pybamm.lithium_ion.SPM({"SEI": "electron-migration limited"})}
# creating a BatchStudy object with the given experimen, model and parameter_values
batch_study = pybamm.BatchStudy(
models=model,
experiments=experiment,
parameter_values=parameter_values,
permutations=True,
)
# solving and plotting the result
batch_study.solve(initial_soc=1)
labels = [
f"Inner SEI open-circuit potential [V]: {inner_sei_oc_v}"
for inner_sei_oc_v in inner_sei_oc_v_values
]
batch_study.plot(labels=labels)
2022-07-26 16:44:42.414 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/10 (35.637 ms elapsed) --------------------
2022-07-26 16:44:42.415 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:42.565 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:42.594 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:42.684 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:42.726 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:42.921 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/10 (542.652 ms elapsed) --------------------
2022-07-26 16:44:42.922 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:43.047 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:43.063 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:43.128 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:43.142 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:43.234 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/10 (855.739 ms elapsed) --------------------
2022-07-26 16:44:43.235 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:43.356 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:43.371 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:43.435 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:43.449 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:43.554 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/10 (1.175 s elapsed) --------------------
2022-07-26 16:44:43.554 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:43.676 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:43.691 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:43.756 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:43.769 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:44.037 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/10 (1.658 s elapsed) --------------------
2022-07-26 16:44:44.037 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:44.171 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:44.187 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:44.255 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:44.270 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:44.374 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/10 (1.995 s elapsed) --------------------
2022-07-26 16:44:44.374 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:44.503 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:44.518 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:44.588 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:44.601 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:44.710 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/10 (2.331 s elapsed) --------------------
2022-07-26 16:44:44.710 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:44.836 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:44.852 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:44.922 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:44.935 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:45.033 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/10 (2.655 s elapsed) --------------------
2022-07-26 16:44:45.034 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:45.156 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:45.171 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:45.238 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:45.252 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:45.369 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/10 (2.991 s elapsed) --------------------
2022-07-26 16:44:45.370 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:45.512 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:45.529 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:45.596 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:45.610 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:45.711 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/10 (3.333 s elapsed) --------------------
2022-07-26 16:44:45.712 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:45.849 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:45.864 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:45.932 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:45.947 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:46.055 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 3.333 s
2022-07-26 16:44:46.893 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/10 (36.421 ms elapsed) --------------------
2022-07-26 16:44:46.894 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:47.052 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:47.080 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:47.172 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:47.219 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:47.398 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/10 (542.151 ms elapsed) --------------------
2022-07-26 16:44:47.399 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:47.522 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:47.690 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:47.760 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:47.774 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:47.872 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/10 (1.016 s elapsed) --------------------
2022-07-26 16:44:47.873 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:48.023 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:48.040 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:48.115 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:48.133 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:48.234 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/10 (1.378 s elapsed) --------------------
2022-07-26 16:44:48.234 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:48.365 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:48.379 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:48.446 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:48.460 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:48.557 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/10 (1.700 s elapsed) --------------------
2022-07-26 16:44:48.557 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:48.688 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:48.704 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:48.776 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:48.790 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:48.887 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/10 (2.031 s elapsed) --------------------
2022-07-26 16:44:48.887 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:49.019 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:49.034 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:49.102 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:49.116 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:49.209 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/10 (2.353 s elapsed) --------------------
2022-07-26 16:44:49.210 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:49.335 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:49.350 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:49.416 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:49.430 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:49.533 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/10 (2.677 s elapsed) --------------------
2022-07-26 16:44:49.534 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:49.658 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:49.673 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:49.740 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:49.755 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:49.851 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/10 (2.995 s elapsed) --------------------
2022-07-26 16:44:49.852 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:49.977 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:49.992 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:50.065 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:50.078 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:50.176 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/10 (3.320 s elapsed) --------------------
2022-07-26 16:44:50.176 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:50.307 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:50.324 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:50.420 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:50.445 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:50.542 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 3.320 s
2022-07-26 16:44:51.360 - [NOTICE] callbacks.on_cycle_start(174): Cycle 1/10 (36.824 ms elapsed) --------------------
2022-07-26 16:44:51.360 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:51.515 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:51.545 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:51.638 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:51.684 - [NOTICE] callbacks.on_step_start(182): Cycle 1/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:52.031 - [NOTICE] callbacks.on_cycle_start(174): Cycle 2/10 (707.971 ms elapsed) --------------------
2022-07-26 16:44:52.031 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:52.156 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:52.171 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:52.238 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:52.251 - [NOTICE] callbacks.on_step_start(182): Cycle 2/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:52.355 - [NOTICE] callbacks.on_cycle_start(174): Cycle 3/10 (1.033 s elapsed) --------------------
2022-07-26 16:44:52.356 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:52.480 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:52.496 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:52.563 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:52.577 - [NOTICE] callbacks.on_step_start(182): Cycle 3/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:52.676 - [NOTICE] callbacks.on_cycle_start(174): Cycle 4/10 (1.353 s elapsed) --------------------
2022-07-26 16:44:52.676 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:52.800 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:52.815 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:52.881 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:52.895 - [NOTICE] callbacks.on_step_start(182): Cycle 4/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:52.989 - [NOTICE] callbacks.on_cycle_start(174): Cycle 5/10 (1.666 s elapsed) --------------------
2022-07-26 16:44:52.990 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:53.114 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:53.129 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:53.196 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:53.210 - [NOTICE] callbacks.on_step_start(182): Cycle 5/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:53.308 - [NOTICE] callbacks.on_cycle_start(174): Cycle 6/10 (1.985 s elapsed) --------------------
2022-07-26 16:44:53.308 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:53.432 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:53.447 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:53.517 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:53.531 - [NOTICE] callbacks.on_step_start(182): Cycle 6/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:53.627 - [NOTICE] callbacks.on_cycle_start(174): Cycle 7/10 (2.305 s elapsed) --------------------
2022-07-26 16:44:53.628 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:53.753 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:53.768 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:53.836 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:53.849 - [NOTICE] callbacks.on_step_start(182): Cycle 7/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:53.945 - [NOTICE] callbacks.on_cycle_start(174): Cycle 8/10 (2.622 s elapsed) --------------------
2022-07-26 16:44:53.945 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:54.072 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:54.086 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:54.160 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:54.173 - [NOTICE] callbacks.on_step_start(182): Cycle 8/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:54.269 - [NOTICE] callbacks.on_cycle_start(174): Cycle 9/10 (2.946 s elapsed) --------------------
2022-07-26 16:44:54.269 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:54.392 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:54.413 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:54.484 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:54.498 - [NOTICE] callbacks.on_step_start(182): Cycle 9/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:54.603 - [NOTICE] callbacks.on_cycle_start(174): Cycle 10/10 (3.281 s elapsed) --------------------
2022-07-26 16:44:54.604 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 1/5: Discharge at C/10 for 10 hours or until 3.3 V
2022-07-26 16:44:54.731 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 2/5: Rest for 1 hour
2022-07-26 16:44:54.747 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 3/5: Charge at 1 A until 4.1 V
2022-07-26 16:44:54.816 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 4/5: Hold at 4.1 V until 50 mA
2022-07-26 16:44:54.829 - [NOTICE] callbacks.on_step_start(182): Cycle 10/10, step 5/5: Rest for 1 hour
2022-07-26 16:44:54.926 - [NOTICE] callbacks.on_experiment_end(222): Finish experiment simulation, took 3.281 s
[6]:
<pybamm.plotting.quick_plot.QuickPlot at 0x7ffdea372580>
The difference in the individual plots is not very well visible in the above slider plot, but we can access all the simulations created by BatchStudy
(batch_study.sims
) and pass it to pybamm.plot_summary_variables
to plot the summary variables (more details on “summary variables” are available in the `simulating-long-experiments
<./simulations_and_experiments/simulating-long-experiments.ipynb>`__ notebook).
Comparing summary variables#
[7]:
pybamm.plot_summary_variables([sim.solution for sim in batch_study.sims])
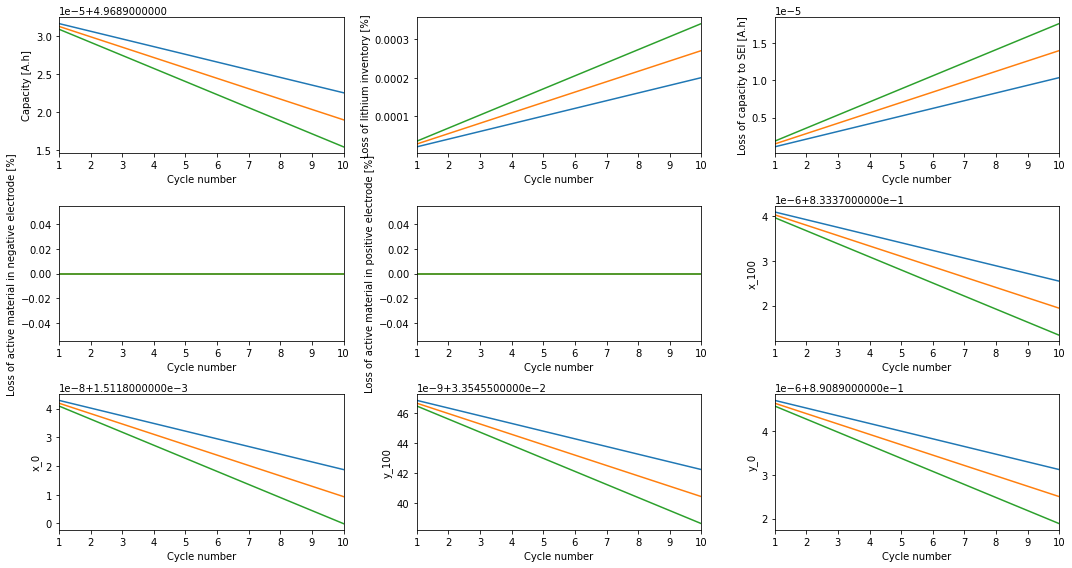
[7]:
array([[<AxesSubplot:xlabel='Cycle number', ylabel='Capacity [A.h]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of lithium inventory [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of capacity to SEI [A.h]'>],
[<AxesSubplot:xlabel='Cycle number', ylabel='Loss of active material in negative electrode [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='Loss of active material in positive electrode [%]'>,
<AxesSubplot:xlabel='Cycle number', ylabel='x_100'>],
[<AxesSubplot:xlabel='Cycle number', ylabel='x_0'>,
<AxesSubplot:xlabel='Cycle number', ylabel='y_100'>,
<AxesSubplot:xlabel='Cycle number', ylabel='y_0'>]], dtype=object)
Other than the above examples, the BatchStudy
class can be used to compare a lot of different configurations, like models with different SEIs or a model with reversible and irreversible lithium plating, etc.
References#
The relevant papers for this notebook are:
[8]:
pybamm.print_citations()
[1] Joel A. E. Andersson, Joris Gillis, Greg Horn, James B. Rawlings, and Moritz Diehl. CasADi – A software framework for nonlinear optimization and optimal control. Mathematical Programming Computation, 11(1):1–36, 2019. doi:10.1007/s12532-018-0139-4.
[2] Chang-Hui Chen, Ferran Brosa Planella, Kieran O'Regan, Dominika Gastol, W. Dhammika Widanage, and Emma Kendrick. Development of Experimental Techniques for Parameterization of Multi-scale Lithium-ion Battery Models. Journal of The Electrochemical Society, 167(8):080534, 2020. doi:10.1149/1945-7111/ab9050.
[3] Marc Doyle, Thomas F. Fuller, and John Newman. Modeling of galvanostatic charge and discharge of the lithium/polymer/insertion cell. Journal of the Electrochemical society, 140(6):1526–1533, 1993. doi:10.1149/1.2221597.
[4] Charles R. Harris, K. Jarrod Millman, Stéfan J. van der Walt, Ralf Gommers, Pauli Virtanen, David Cournapeau, Eric Wieser, Julian Taylor, Sebastian Berg, Nathaniel J. Smith, and others. Array programming with NumPy. Nature, 585(7825):357–362, 2020. doi:10.1038/s41586-020-2649-2.
[5] Scott G. Marquis, Valentin Sulzer, Robert Timms, Colin P. Please, and S. Jon Chapman. An asymptotic derivation of a single particle model with electrolyte. Journal of The Electrochemical Society, 166(15):A3693–A3706, 2019. doi:10.1149/2.0341915jes.
[6] Peyman Mohtat, Suhak Lee, Jason B Siegel, and Anna G Stefanopoulou. Towards better estimability of electrode-specific state of health: decoding the cell expansion. Journal of Power Sources, 427:101–111, 2019.
[7] Peyman Mohtat, Suhak Lee, Valentin Sulzer, Jason B. Siegel, and Anna G. Stefanopoulou. Differential Expansion and Voltage Model for Li-ion Batteries at Practical Charging Rates. Journal of The Electrochemical Society, 167(11):110561, 2020. doi:10.1149/1945-7111/aba5d1.
[8] Valentin Sulzer, Scott G. Marquis, Robert Timms, Martin Robinson, and S. Jon Chapman. Python Battery Mathematical Modelling (PyBaMM). Journal of Open Research Software, 9(1):14, 2021. doi:10.5334/jors.309.
[9] Pauli Virtanen, Ralf Gommers, Travis E. Oliphant, Matt Haberland, Tyler Reddy, David Cournapeau, Evgeni Burovski, Pearu Peterson, Warren Weckesser, Jonathan Bright, and others. SciPy 1.0: fundamental algorithms for scientific computing in Python. Nature Methods, 17(3):261–272, 2020. doi:10.1038/s41592-019-0686-2.